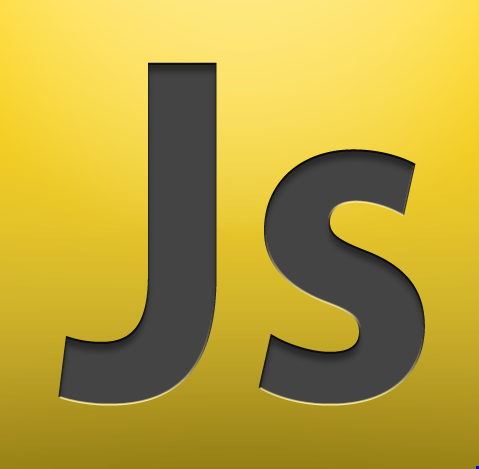
Published: 03-08-2016
Overview
Well, it's not like I can explain these patterns better than anyone else. This stuff is really just a personal reference so I can quickly remind myself sometimes. Much more comprehensive literature can be found in the references at the bottom. Lots of this stuff is from those references.
Constructor Pattern
So, in JavaScript calling a function prefixed with the new keyword turns it into a constructor. The new keyword also acts to bind this to the object created.
function Aircraft( make, year, cycles ) {
this.make = make;
this.year = year;
this.cycles = cycles;
this.getInfo = function () {
return "The " + this.make + " has done " + this.cycles + " cycles since " + this.year;
}
}
var my_aircraft = new Aircraft( 'Boeing 747', 1999, 1000 );
console.log( my_aircraft.getInfo() )
// The Boeing 747 has done 1000 cycles since 1999
People don't seem to like using the new keyword though, it ruffles a few feathers. One argument is that if you forget the new bad things happen (stuff gets put on the global scope). Consistency is key I guess, if others use your library, make sure they know whether to use new or not, and keep it the same throughout. You can always add in a bit of code to prevent bad stuff from happening (taken from [4]). It basically checks whether this has been bound to the object add throws an error if not. It makes sure the function can only be used as a constructor.
function Aircraft() {
if (!(this instanceof Aircraft)) {
throw new Error("Aircraft needs to be called with 'new'");
}
}
var my_aircraft = Aircraft();
// Error: Aircraft needs to be called with 'new'
With Prototypes
The constructor pattern is often augmented with prototypes. Methods are added to the constructor's prototype so they don't have to be added to each object individually, saving memory. All objects made by the constructor point to the same prototype enabling re-use. The object will only delegate to the prototype should the method not be available on the object. This is only really needed when making a lot of the same objects. Why and where does the prototpe come from? All functions get a public property on them called prototype. When called as a constructor the returned object is prototype-linked to the function's prototype.
function Aircraft( make, build_date ) {
this.make = make;
this.build_date = build_date;
}
Aircraft.prototype.getInfo = function () {
return "The " + this.make + " has been flying since " + this.build_date;
}
var my_aircraft = new Aircraft( 'Boeing 747', 1999 );
console.log( my_aircraft.getInfo() )
// The Boeing 747 has done 1000 cycles since 1999
This kind of functionality is used to provide 'class like' behaviour in JavaScript. It's this kind-of misunderstood, fake class behaviour, that seems to annoy some people. It's not a 'classical OOP class' because when we call new we get a new object, but every object delegates back to the same prototype. Classical 'Classes' are a blue prints that are copied to new objects, not referenced. In a way JavaScript is more powerful because we can dynamically change our fake 'class' or objects (they're not really classes). This, however, also makes it more dangerous.
With Prototypal Inheritance
People try to reproduce classical 'class inheritance' by linking prototypes. This is called prototypal inheritance. Below is an example where a new 'class' FighterJet inherits from Aircraft using this method.
function Aircraft( make, build_date ) {
this.make = make;
this.build_date = build_date;
}
Aircraft.prototype.getInfo = function () {
return "The " + this.make + " has been flying since " + this.build_date;
}
function FighterJet(make, build_date, missiles) {
Aircraft.call( this, make, build_date );
this.missiles = missiles;
}
// Make a new 'FighterJet.prototype' and link it 'Aircraft.prototype'
FighterJet.prototype = Object.create( Aircraft.prototype );
FighterJet.prototype.getFighterInfo = function () {
return 'It can carry ' + this.missiles + ' missiles.'
}
var fighter_aircraft = new FighterJet( 'F22', 2005, 10 );
console.log( fighter_aircraft.getInfo() )
console.log( fighter_aircraft.getFighterInfo() )
// The F22 has been flying since 2005
// It can carry 10 missiles.
// Side effect --------------------------------------------------//
// Broke the FighterJet constructor property
console.log(fighter_aircraft.constructor === FighterJet) // False
The code above has tried to replicate some classical class inheritance and polymorphism. The child class inherits the getInfo method. The child class also overrides the parents constructor call (a type of polymorphism). Object.create is used to create a new object for FighterJet.prototype whose prototype-link points to Aircraft.prototype. One problem with this is that it destroys the FighterJet.prototype.constructor property. This needs to be fixed if relying on the constructor property for identification. Visually, the code above looks like this:
ES6
This method of imitating class inheritance is kind-of how ES6 does it (not exactly). ES6 introduces the class and super keywords which are essentially just syntactic sugar for this pattern. Using typescript and ES6 'classes' to implement the same functionality one can see this in action:
class Aircraft {
make;
build_date;
constructor( make, build_date ) {
this.make = make;
this.build_date = build_date;
}
getInfo () {
return "The " + this.make + " has been flying since " + this.build_date;
}
}
class FighterJet extends Aircraft {
missiles;
constructor( make, build_date, missiles ) {
super( make, build_date )
this.missiles = missiles;
}
getFighterInfo() {
return 'It can carry ' + this.missiles + ' missiles.'
}
}
let fighter_aircraft = new FighterJet( 'F22', 2005, 10 );
console.log( fighter_aircraft.getInfo() )
console.log( fighter_aircraft.getFighterInfo() )
var Aircraft = (function () {
function Aircraft(make, build_date) {
this.make = make;
this.build_date = build_date;
}
Aircraft.prototype.getInfo = function () {
return "The " + this.make + " has been flying since " + this.build_date;
};
return Aircraft;
}());
var FighterJet = (function (_super) {
__extends(FighterJet, _super);
function FighterJet(make, build_date, missiles) {
_super.call(this, make, build_date);
this.missiles = missiles;
}
FighterJet.prototype.getFighterInfo = function () {
return 'It can carry ' + this.missiles + ' missiles.';
};
return FighterJet;
}(Aircraft));
var __extends = (this && this.__extends) || function (d, b) {
for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p];
function __() { this.constructor = d; }
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
};
var fighter_aircraft = new FighterJet('F22', 2005, 10);
console.log(fighter_aircraft.getInfo());
console.log(fighter_aircraft.getFighterInfo());
It looks a little different because Typescript wraps the functions in IIFEs. Imagine the code after the IIFEs execute and you can see that it is pretty much identical to the pattern above. The only difference is the use of __extend instead of a plain Object.create. In this situation they do the same thing. All that extra stuff is to handle:
1) The problem of destroying the constructor - The constructor is replaced using this.constructor = d
2) To accommodate static methods - Static methods have to be copied across to the 'inheriting class' because they are called by Class.staticMethod(), not from an instantiated object.
Anyway, faking classes is interesting. It might be more work that its worth but if the programmer is used to using classical classes it might suit their thought process. Some JavaScript purists on the other hand tend to frown upon this. "You're not using the language's strengths, you're trying to make it feel like a class-based language, which it isn't", is the general notion. They then point you in this direction, the Pure Prototype Pattern, or Behaviour Delegation, or OLOO (Objects Linked to Other Objects) as coined by Kyle Simpson.
Pure Prototype Pattern or Behaviour Delegation
This pattern works to JavaScript strengths. This pattern makes use of Object.create(). The pattern is based around creating an object and setting it's prototype-link to an object of our choosing. This provides a way to delegate behaviour up a chain. Some books describe the Prototype Pattern more generally, as anything with Prototype (combined patterns). Here I mean linking objects, not linking object's prototypes as seen in the 'Constructor with Linked Prototypes' example. An example is shown below.
var Aircraft = {
init: function ( make, build_date ) {
this.make = make;
this.build_date = build_date;
},
getInfo: function () {
return "The " + this.make + " has been flying since " + this.build_date;
}
}
var FighterJet = Object.create( Aircraft );
FighterJet.hasMissiles = function (missiles) {
this.missiles = missiles;
}
FighterJet.getFighterInfo = function () {
return "It can carry " + this.missiles + " missiles.";
}
var fighter_aircraft = Object.create( FighterJet );
fighter_aircraft.init( 'F22', '2005' );
fighter_aircraft.hasMissiles(10);
console.log( fighter_aircraft.getInfo() );
console.log( fighter_aircraft.getFighterInfo() );
// The F22 has been flying since 2005
// It can carry 10 missiles.
With this pattern there is no polymorphism and so no weird Function.call()s. This simple example doesn't really do it justice, but it does tend to simplify the thought process. Function names are likely to be more specific and less generic. This is because they aren't 'shared' polymorphically between 'classes'. There are however more lines of code to create the fighter_jet object, mainly due to the separation of object creation and initialisation.
Module Pattern
'Modules are all around us, they're everywhere we go'. Modules are used to separate and organise code. They can be created wrapping code with an IIFE (Immediately-Invoked Function Expression). The IIFE provides the code with its own scope, thus it doesn't pollute the global scope. They have a bunch of variations to help make variables and functions 'private'. Some argue this makes testing hard, but that depends on what you testing focus is (Unit vs Behaviour etc.).
var myNamespace = (function () {
var privateVar = 0,
privateMethod;
privateMethod = function ( something ) {
console.log( something );
}
return {
publicVar: "Boo",
publicFunction: function ( something ) {
privateVar++;
privateMethod( something )
},
publicFunction2: function ( something ) {
privateVar++;
namespace.publicFunction( something );
}
}
}) ();
Modules are also implemented in other ways such as: CommmonJs modules, AMD modules, Object literal notion and ES2015 modules. The previous pattern is written like this with CommonJS:
// code.js Export file
var privateVar = 0,
privateMethod;
privateMethod = function ( something ) {
console.log( something );
}
module.exports = {
publicVar: "Boo",
publicFunction: function ( something ) {
privateVar++;
privateMethod( something )
},
publicFunction2: function ( something ) {
privateVar++;
namespace.publicFunction( something );
}
}
// Import file
var myNamespace = require('code.js')
CommonJs takes care of the scoping and the IIFE is no longer required.
Revealing Module Pattern
The revealing module pattern exposes a public API by referencing functions.
var myRevealingModule = (function () {
var privateVar = 'Thomas',
publicVar = 'Boo';
function privateFunction() {
console.log('Name: ' + 'privateVar');
}
function publicSetname( strName ) {
privateVar = strName;
}
function publicGetName(argument) {
privateFunction();
}
return {
setName: publicSetname,
greeting: publicVar,
getName: publicGetName
}
}) ();
Observer Pattern
To come
Singleton Pattern
To come