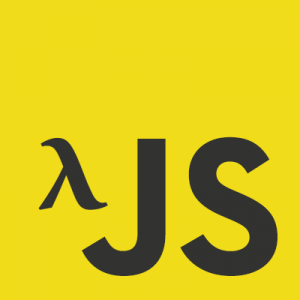
Published: 09-03-2016
Pure and Impure functions
Functional programming requires pure functions. Pure functions are functions that have no so called 'side effects'. They don't change state somewhere outside of the function. Often a functional program with have to include some impure functions as a necessary evil, for example I/O. The impure functions are often not publicly exposed and do stuff 'behind the scenes'.
Example of an impure function:
function foo (x) {
y = x * 2;
z = x * 3;
}
var y, z;
foo(5);
y; // 10
z; // 15
Here state outside the called function (global variables) are changed when the foo function is run. The reason impure functions and state changes are frowned upon is because it makes code hard to reason about. This doesn't mean stateful and impure programs are bad. They're just a different way of solving a program. Below is an example of wrapping a function to make it pure.
function foo (x) {
y = y * x;
z = y * x;
}
var y = 2, z = 3;
foo(5);
y; // 10
z; // 15
foo(5);
y; // 50
z; // 75
function bar (x,y,z) {
foo(x);
return [y,z];
function foo (x) {
y = y * x;
z = y * x;
}
}
bar(5,2,3); // [10,15]
bar(5,10,15); // [50,75]
In the pure function the all the state is passed into the function. And so every time the function is called with the same inputs the result will be the same. As multiple values cannot be returned they have to be wrapped up in a container, in this case and array. The function is essensially returning new state which could be used by another function or just discarded. Instead of wrapping the foo function it could have been re-factored to make it pure.
Composition
Composition is concerned with taking the output of one function and using it as the input of another function. It could also be part of the input rather than the whole input (currying). a simple example of combining functions can be seen below. This example is however impure as the intermediate state is being held in a variable.
function sum (x,y) {
return x + y;
}
function mult (x,y) {
return x * y;
}
// 5 + (3 * 4)
var z = mult(3,4);
z = sum(z,5);
z; // 17
From the perspective of our program we can make it pure by passing the result directly into the second function:
sum( mult(3,4), 5 ); // 17
Above is an example of manual compilation. We could create a function that programmatically composes two functions. The example below shows a possible way of doing this:
function sum(x,y) {
return x + y;
}
function mult(x,y) {
return x * y;
}
function compose2 (fn1, fn2) {
return function comp () {
var args = [].slice.call(arguments);
return fn2(
fn1(args.shift(), args.shift()),
args.shift()
);
}
}
var multAndSum = compose2(mult, sum);
console.log(multAndSum(3,4,5)); // 17
This composition makes a bunch of assumptions about how the functions work. It would therefore not be particularly useful in other scenarios. There are however a lot of common scenarios faced in composition and this is where patterns with funky names like functors and monads come in.
Immutability
Immutability is an important component of functional programming. There are a few misconceptions about immutability in javascript so just to make it clears here are some examples:
var x = 2;
x++; // allowed
const y = 3;
y ++; // not allowed
const z = [4,5,6];
z = 10; // not allowed
z[0] = 10; // allowed
const w = Object.freeze([4,5,6]);
w = 10; // not allowed
w[0] = 10; // not allowed
People sometimes refer to const making as a immutable variable which is wrong and right. The keyword const actually makes the assignment or binding immutable, not the actual value. This is demonstrated in the example above. Primative values by there nature are immutable but arrays and objects are bound by reference. Using the keyword const makes this reference immutable but not the actual value.
The utility Object.Freeze() provides shallow immutability on an object. This means all properties or indices (for arrays) up to one level deep are immutable but no further. The real point is to develop the discipline to not mutate value regardless of whether the language lets you or not. Mutating an input value is essentially creating a side effect. Instead creating a copy or a new set of values is good practise. Below is and example of mutating and not mutating the input. Mutating the input in this case causing a side effect (impure function) as arrays are passed by reference in javascript not by value like primitives.
function doubleThemMutable (list) {
for (var i = 0; i < list.length; i++) {
list[i] = list[i] * 2;
}
}
var arr = [3,4,5];
doubleThemMutable(arr);
arr; // [6,8,10]
function doubleThemImmutable (list) {
var newList = [];
for (var i = 0; i < list.length; i++) {
newList[i] = list[i] * 2;
}
return newList;
}
var arr = [3,4,5];
doubleThemImmutable(arr);
arr; // [6,8,10]
Closure
In very basic terms, Closure is when a function 'remembers' the variables around it even when that function is executed elsewhere. Below is a simple example of closure:
function foo () {
var count = 0;
return function () {
return count++;
};
}
var x = foo();
x(); // 1
x(); // 2
x(); // 3
Normally one might think that foo()'s internal state would disappear after it has finished executing, before x() is called. The reason this doesn't happen is because the inner function has closure over the variable count by referencing it. The scope or state is kept because of this reference. This is not very functional use of closure as count changes over time and doesn't maintain its state. Closure applied in a more functional way might look like:
function sumX (x) {
return function (y) {
return x + y;
};
}
var add10 = sumX(10);
add10(3); // 13
add10(14); // 24
Here the returned function still has closure over x but x never changes state. Currying uses this technique to take functions that require multiple inputs/variables and presets some of them using closure.
Recursion
Recursion is favoured over iteration in functional programming. A loop generally makes use of a counter which is considered a side effect. A recursive function calls itself as part of the solution. It has a base case which stops the recursion. Recursion can also be mutual when two or more functions call each other until they reach a base case.
In practice recursive functions can cause some problems as a result of creating too many stack frames. To prevent running out of memory there is an arbitrary limit to the depth of the call stack, around 10,000 in most javascript engines. ES6 partially solves this problem by introducing proper tail calls. A tail call is when the function call is made and returned as the last statement in the function. This lets the compiler know that it can reuse the stack frame rather than creating a new one.
Below is a comparison of using a loop and recusion to sum up all the arguments passed as an array into a function:
function sumIter (arr) {
var sum = 0;
for (var i=0; i < arr.length; i++) {
sum = sum + arr[i];
}
return sum;
}
sumIter([3,4,5]) // 12
function sumRecur (arr) {
if (arr.length <= 2) {
return arr[0] + arr[1];
}
return (
arr[0] +
sumRecur(arr.slice(1))
);
}
sumRecur([3,4,5]) // 12
List transformation (map)
In functional programming often operations are expressed as a list operations. The list doesn't necessary have to contain many values, it could contain 1 or even none. As a pure operation it doesn't matter how many items are in the list. It also doesn't matter what order they are done in. A transformation is taking one value and doing something to it so that you get a different value out.
The type of pure transformations that can be defined are fairly limitless. Common examples are often on numbers and involve mathematical operations but they could be on strings, functions etc. A transformation or mapping always returns a list with the same number of items, transformed. A simple example is shown below:
function doubleIt (v) {
return v * 2;
}
function transform (arr,fn) {
var list = [];
for (var i=0; i<arr.length; i++) {
list[i] = fn(arr[i]);
}
return list;
}
transform([1,2,3,4,5], doubleIt);
// [2,4,6,8,10]
Notice a new array is created to store the transformed values, applying the immutability principle. Instead of writing our own utility like this we can use the one built into javascript .map().
function doubleIt (v) {
return v * 2;
}
[1,2,3,4,5].map(doubleIt);
// [2,4,6,8,10]
This utility is added as a method on the prototype. The net result is that composing transformation function has to be done by chaining when using the built it .map(). This is different to what a 'traditional' functional programmer might do. Instead of composing the actual operations and performing the map once the map must be re-performed for each operation.
List Exclusion (filter)
This is used when you want to exclude or filter out items from a list. As an example, a filter which keeps odd numbers is shown below. The function isOdd() returns true when the number is odd cause the item to be added to the returned array.
function isOdd (v) {
return v % 2 == 1;
}
function exclude (arr,fn) {
var list = [];
for (var i = 0; i < arr.length; i++) {
if (fn(arr[i])) {
list.push(arr[i]);
}
}
return list;
}
exclude([1,2,3,4,5], isOdd);
// [1,3,5]
Javascript's built in utility for exclusion is .filter().
function isOdd (v) {
return v % 2 == 1;
}
[1,2,3,4,5].filter(isOdd);
// [1,3,5]
List Composition (reduce)
This deals with composition of items within a list. An example might be to take a list of numbers and add them all together. Reduce is a little bit more complicated than map or filter. It is also actually possible to use reduce in a non pure way.
function mult (previous,current) {
return previous * current;
}
function compose (arr,fn,initial) {
var total = initial;
for (var i = 0; i < arr.length; i++) {
total = fn(total,arr[i]);
}
return total;
}
compose([1,2,3,4,5],mult,1);
// 20
Javascript's built in utility for this is .reduce(). This method also provides a few extra arguments to the callback function that are not used here.
function mult (previous,current) {
return previous * current;
}
[1,2,3,4,5].reduce(mult,1)
// 20
List Iteration
The only reason a list iteration would be useful is to change the list itself or something outside the function. These are side effects and so make it impure. This doesn't matter if you need to do some impure like logging to the console etc. An example is shown below:
function logValue (v) {
console.log(v);
}
function iterate (arr,fn) {
for (var i = 0; i < arr.length; i++) {
fn(arr[i]);
}
}
iterate([1,2,3,4,5],logValue);
Javascript also has a built in method for this, .forEach()
function logValue (v) {
console.log(v);
}
[1,2,3,4,5].forEach(logValue)
Future reading